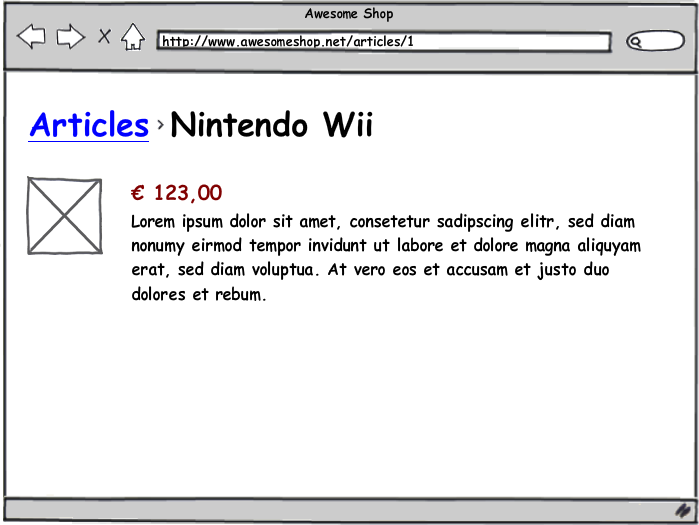
"Cat Burglar" by Amber Grundy
http://www.flickr.com/photos/abear23/1444321123/
We are looking for new colleagues!
As a customer
in order to gather the products I want to purchase
I want to add items to my shopping cart
Scenario: add an item to the shopping cart Given an article with the name "Nintendo Wii" When I am on the homepage And I follow "Nintendo Wii" And I press "Add to cart" Then I should see "Item was added to cart"
Scenario: add an item to the shopping cart Given an article with the name "Nintendo Wii" When I am on the homepage And I follow "Nintendo Wii" And I press "Add to cart" Then I should see "Item was added to cart"
Scenario: add an item to the shopping cart Given an article with the name "Nintendo Wii" When I am on the homepage And I follow "Nintendo Wii" And I press "Add to cart" Then I should see "Item was added to cart" Unknown class "Article" 1 scenario (1 failed)
class Article attr_accessor :name end
Scenario: add an item to the shopping cart Given an article with the name "Nintendo Wii" When I am on the homepage And I follow "Nintendo Wii" And I press "Add to cart" Then I should see "Item was added to cart" Error retrieving page (404 not found) 1 scenario (1 failed)
Scenario: add an item to the shopping cart Given an article with the name "Nintendo Wii" When I am on the homepage And I follow "Nintendo Wii" And I press "Add to cart" Then I should see "Item was added to cart" No such link "Nintendo Wii" 1 scenario (1 failed)
Scenario: add an item to the shopping cart Given an article with the name "Nintendo Wii" When I am on the homepage And I follow "Nintendo Wii" And I press "Add to cart" Then I should see "Item was added to cart" No such button "Add to cart" 1 scenario (1 failed)
Scenario: add an item to the shopping cart Given an article with the name "Nintendo Wii" When I am on the homepage And I follow "Nintendo Wii" And I press "Add to cart" Then I should see "Item was added to cart" Expected to see "Item was added to cart" 1 scenario (1 failed)
Scenario: add an item to the shopping cart Given an article with the name "Nintendo Wii" When I am on the homepage And I follow "Nintendo Wii" And I press "Add to cart" Then I should see "Item was added to cart" 1 scenario (1 passed)
writing software != building bridges
View the rest of this chapter here:
why-expanded.with-fonts.pdf
Scenario: add an item to the shopping cart Given an article with the name "Nintendo Wii" When I am on the homepage And I follow "Nintendo Wii" And I press "Add to cart" Then I should see "Item was added to cart"
describe Hero do describe '#attack' do it "should damage the target for the hero's strength" do monster = Monster.create(:hitpoints => 100) hero = Hero.create(:strength => 70) hero.attack(monster) monster.hitpoints.should == 30 end end end
visit '/login' fill_in 'E-mail', :with => 'user@example.com' fill_in 'Password', :with => 'secret' click_link 'Sign in' page.should have_content('Signed in successfully')
mysqldump -uroot -psecret -d hero_development |↲
mysql -uroot -psecret hero_test
"The RSS feed should include the latest forum comment"
describe Feed, '.to_rss' do it "should include the latest forum comment" do forum = Forum.create(:name => "Example Forum") user = User.create(:email => "foo@bar.com", :name => "Hans Dampf") topic = Topic.create(:forum => forum, :subject => "Example Topic", :author => user) Comment.create(:topic => topic, :author => user, :text => "Hi world!") Feed.to_rss.should include("Hi world!") end end
User.blueprint do name "John Doe" email "john@doe.com" password 'secret' end Forum.blueprint do name "Example Forum" end Topic.blueprint do forum subject "Example Topic" end Comment.blueprint do topic author text "Lorem ipsum dolor sit amet" end
describe Feed, '.to_rss' do it "should include the latest forum comment" do Comment.make(:text => "Hi world!") Feed.to_rss.should include("Hi world!") end end
PayPal.capture_payment("RE-23400123") GoogleMaps.fetch_geocode("Augsburg") NuclearMissile.new.launch system("rm -rf /")
class Order def initialize(number) @number = number @paid = false end def finish if PayPal.capture_payment(@number) @paid = true end end def paid? @paid end end
describe Order, '#finish' do
it "should mark the order as paid" do
order = Order.new("ON-5512")
order.finish
order.should be_paid
end
end
Order finish should mark the order as paid FAILED
describe Order, '#finish' do
it "should mark the order as paid" do
order = Order.new("ON-5512")
order.finish
order.should be_paid
end
end
Order finish should mark the order as paid FAILED
PayPalError: Unknown transaction reference "ON-5512"
1 example, 1 failure
describe Order, '#finish' do
it "should mark the order as paid" do
order = Order.new("ON-5512")
order.finish
order.should be_paid
end
end
Order finish should mark the order as paid FAILED
HTTPError: Could not resolve host api.paypal.com
1 example, 1 failure
describe Order, '#finish' do it "should mark the order as paid" do PayPal.stub(:capture_payment).and_return(true) order = Order.new("ON-5512") order.finish order.should be_paid end end 1 example, 0 failures Order finish should mark the order as paid FAILED
describe Order, 'finish' do it "should capture the payment through PayPal" do # ??? end end
describe Order, 'finish' do it "should capture the payment through PayPal" do PayPal .should_receive(:capture_payment)↲ .with("ON-5512")↲ .and_return(true) order = Order.new("ON-5512") order.finish end end
Got interested to work with the good guys?
Talk to me!